
Engineering, 04.04.2020 09:35 McMingMing
In this lab, you will be creating a class that implements the Rule of Three (A Destructor, A Copy Constructor, and a Copy Assignment Operator). You are to create a program that prompts users to enter in contact information, dynamically create each object, then print the information of each contact to the screen. Some code has already been provided for you. To receive full credit make sure to implement the following:
Default Constructor - set Contact id to -1
Overloaded Constructor - used to set the Contact name, phoneNumber and id (Should take in 3 parameters)
Destructor
Copy Constructor
Copy Assignment Operator
Any other useful functions (getters/setters)
Main. cpp
#include
#include
#include "Contact. h"
using namespace std;
int main() {
const int NUM_OF_CONTACTS = 3;
vector contacts;
for (int i = 0; i < NUM_OF_CONTACTS; i++) {
string name, phoneNumber;
cout << "Enter a name: ";
cin >> name;
cout << "Enter a phoneNumber; ";
cin >> phoneNumber;
// TODO: Use i, name, and phone number to dynamically create a Contact object on the heap
// HINT: Use the Overloaded Constructor here!
// TODO: Add the Contact * to the vector...
}
cout << "\n\n Contacts \n\n";
// TODO: Loop through the vector of contacts and print out each contact info
// TODO: Make sure to call the destructor of each Contact object by looping through the vector and using the delete keyword
return 0;
}
Contact. h
#ifndef CONTACT_H
#define CONTACT_H
#include
#include
using std::string;
using std::cout;
class Contact {
public:
Contact();
Contact(int id, string name, string phoneNumber);
~Contact();
Contact(const Contact& copy);
Contact& operator=(const Contact& copy);
private:
int *id = nullptr;
string *name = nullptr;
string *phoneNumber = nullptr;
};
#endif
Contact. cpp
#include "Contact. h"
Contact::Contact() {
this->id = new int(-1);
this->name = new string("No Name");
this->phoneNumber = new string("No Phone Number");
}
Contact::Contact(int id, string name, string phoneNumber) {
// TODO: Implement Overloaded Constructor
// Remember to initialize pointers on the heap!
}
Contact::~Contact() {
// TODO: Implement Destructor
}
Contact::Contact(const Contact ©) {
// TODO: Implement Copy Constructor
}
Contact &Contact::operator=(const Contact ©) {
// TODO: Implement Copy Assignment Operator
return *this;
}

Answers: 1

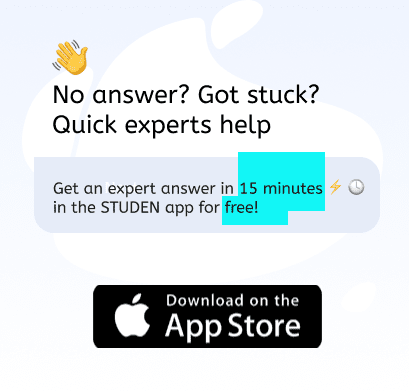
Other questions on the subject: Engineering

Engineering, 04.07.2019 18:10, bckyanne3
Afull journal bearing has a journal diameter of 27 mm, with a unilateral tolerance of -0.028 mm. the bushing bore has a diameter of 27.028 mm and a unilateral tolerance of 0.04 mm. the l/d ratio is 0.5. the load is 1.3 kn and the journal runs at 1200 rev/min. if the average viscosity is 50 mpa-s, find the minimum film thickness, the power loss, and the side flow for the minimum clearance assembly.
Answers: 1


Engineering, 04.07.2019 18:10, oshawn108
Ahot wire operates at a temperature of 200°c while the air temperature is 20°c. the hot wire element is a tungsten wire of 5 um diameter and 2 mm in length. plot using excel current, heat transfer and heat generated by the wire for air velocity varying from 1-10 m/s in steps of lm/s? matlab the sensor voltage output, resistance, or assume nu 0.989 re033pr13 take air properties at tr (200°c20°c)/2 = 110°c properties of tungsten: c 0.13 kj/kg. k 3 p 19250 kg/m k (thermal conductivity) = 174 w/m. k
Answers: 2

Engineering, 04.07.2019 18:20, moneywaydaedae
Air is compressed isentropically from an initial state of 300 k and 101 kpa to a final temperature of 1000 k. determine the final pressure using the following approaches: (a) approximate analysis (using properties at the average temperature) (b) exact analysis
Answers: 1
You know the right answer?
In this lab, you will be creating a class that implements the Rule of Three (A Destructor, A Copy Co...
Questions in other subjects:

Mathematics, 14.04.2020 00:16


Mathematics, 14.04.2020 00:16



History, 14.04.2020 00:16

Mathematics, 14.04.2020 00:16



World Languages, 14.04.2020 00:16