
Computers and Technology, 08.02.2022 01:00 shyshy1791
Hello! I need help with what I should put in my program. cs to test out my code. (my code is below)
What method and code do I write to test it out? Thanks!
namespace DES1_Week1_PlayingCardAssignment
{
public enum Ranks {
Ace = 1,
Two,
Three,
Four,
Five,
Six,
Seven,
Eight,
Nine,
Ten,
Jack,
Queen,
King,
NUM_RANKS
}
public enum Suits {
Hearts = 0,
Diamonds,
Spades,
Clubs,
NUM_SUITS
}
class PlayingCard {
private Suits Suit;
private Ranks Rank;
public PlayingCard(Suits NewSuit, Ranks NewRank) {
Suit = NewSuit;
Rank = NewRank;
}
public static int CompareCards(PlayingCard Card1, PlayingCard Card2) {
int diff = Card2.GetValue() - Card1.GetValue();
if (diff > 0) {
return 1;
} else if (diff < 0) {
return -1;
} else {
return 0;
}
}
public Suits GetSuit() {
return Suit;
}
public Ranks GetRank() {
return Rank;
}
public int GetValue() {
if (Rank == Ranks. Ace) {
return 11;
} else if (Rank == Ranks. King || Rank == Ranks. Queen || Rank == Ranks. Jack) {
return 10;
} else {
return (int)Rank;
}
}
public void (int XPosition, int YPosition) {
char symbol;
if (Suit == Suits. Clubs) {
symbol = ClubSymbol;
} else if (Suit == Suits. Diamonds) {
symbol = DiamondSymbol;
} else if (Suit == Suits. Hearts) {
symbol = HeartSymbol;
} else {
symbol = SpadeSymbol;
}
Console. SetCursorPosition(XPosition, YPosition++);
char[] values = new char[]{ 'A', '2', '3', '4', '5', '6', '7', '8', '9', 'J', 'K', 'Q' };
char value = values[((int)Rank) - 1];
for (int y = 0; y < CardHeight; y++) {
for (int x = 0; x < CardWidth; x++) {
if (x == 0 && y == 0) {
Console. Write("╔");
} else if (x == CardWidth - 1 && y == 0) {
Console. WriteLine("╗");
} else if (x == 0 && y == CardHeight - 1) {
Console. Write("╚");
} else if (x == CardWidth - 1 && y == CardHeight - 1) {
Console. WriteLine("╝");
} else if (x == 0) {
Console. Write("║");
} else if (x == CardWidth - 1) {
Console. WriteLine("║");
} else if ((y == 0 || y == CardHeight - 1) && (x > 0 && x < CardWidth)) {
Console. Write("═");
} else if (x == 1 && y == 1 || (x == CardWidth - 2 && y == CardHeight - 2)) {
Console. Write(value);
} else if (x == CardWidth / 2 && y == CardHeight / 2) {
Console. Write(symbol);
} else {
Console. Write(" ");
}
}
Console. SetCursorPosition(XPosition, YPosition++);
}
}
public int GetAltValue() {
if (Rank == Ranks. Ace) {
return 1;
} else if (Rank == Ranks. King || Rank == Ranks. Queen || Rank == Ranks. Jack) {
return 10;
} else {
return (int)Rank;
}
}
private static char HeartSymbol = Encoding. GetEncoding(437).GetChars(new byte[] { 3 })[0];
private static char DiamondSymbol = Encoding. GetEncoding(437).GetChars(new byte[] { 4 })[0];
private static char ClubSymbol = Encoding. GetEncoding(437).GetChars(new byte[] { 5 })[0];
private static char SpadeSymbol = Encoding. GetEncoding(437).GetChars(new byte[] { 6 })[0];
public static int CardWidth = 5;
public static int CardHeight = 3;
}
}

Answers: 2

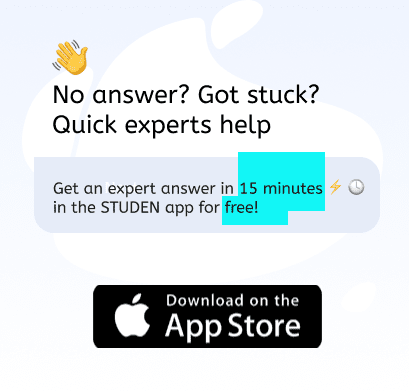
Other questions on the subject: Computers and Technology

Computers and Technology, 22.06.2019 04:50, edenlbarfield
Which are steps taken to diagnose a computer problem? a) reproducing the problem and using error codes b) reproducing the problem and troubleshooting c) using error codes and troubleshooting d) using error codes and stepping functions
Answers: 1

Computers and Technology, 23.06.2019 16:30, isaiahhuettnerowgg8d
What is one reason why indoor air pollution has become an increasing problem.
Answers: 1

Computers and Technology, 24.06.2019 06:50, emmv565628
What are the things you are considering before uploading photos on social media?
Answers: 1
You know the right answer?
Hello! I need help with what I should put in my program. cs to test out my code. (my code is below)...
Questions in other subjects:






Mathematics, 10.12.2020 20:40


Mathematics, 10.12.2020 20:40

English, 10.12.2020 20:40

Physics, 10.12.2020 20:40