
Computers and Technology, 20.08.2021 01:00 mikaelalcool49
Here is the starting code for your assignment. As you can see, so far there is a function to create two random numbers between 1 and 25 and a function to add them together. Your assignment is to create three more functions, add the prototypes correctly, call them from main correctly and output the results. The three functions should be subtractNumbers, multiplyNumbers, and divideNumbers. Remember that division won't always yield an integer so be sure to take care of that issue within the function (don't change the variable declarations).
Don't change the code that I've given you.
Follow the same pattern.
Don't use tools that we haven't studied.
// This program adds, subtracts, multiplies, and divides two random numbers
#include
#include // For rand and srand
#include // For the time function
using namespace std;
// Function declarations
int chooseNumber();
int addNumbers(int, int);
const int MIN_VALUE = 1; // Minimum value
const int MAX_VALUE = 25; // Maximum value
unsigned seed = time(0);
int main()
{
// Seed the random number generator.
srand(seed);
int firstNumber = chooseNumber();
int secondNumber = chooseNumber();
int sum = addNumbers(firstNumber, secondNumber);
cout << "The first number is " << firstNumber << endl;
cout << "The second number is " << secondNumber << endl;
cout << "The sum is " << sum;
return 0;
}
int chooseNumber()
{
// Variable
int number; // To hold the value of the number
number = (rand() % (MAX_VALUE - MIN_VALUE + 1)) + MIN_VALUE;
return number;
}
int addNumbers (int number1, int number2)
{
int number = number1 +number2;
return number;
}
Notice that number is used as a variable in more than one function. This works because number is a variable.

Answers: 2

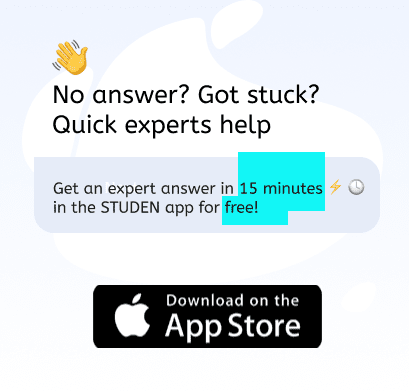
Other questions on the subject: Computers and Technology

Computers and Technology, 23.06.2019 04:10, cvbnkifdfg
2pointswho was mikhail gorbachev? oa. a russian leader who opposed a coupob. a polish leader who founded the labor union "solidarityoc. a soviet leader who called for a closer relationship with the unitedstates, economic reform, and a more open societyd. a soviet leader who called for more oppression in the soviet union
Answers: 3

Computers and Technology, 23.06.2019 19:00, jacobbecker99
Choose the correct citation for the case which established the "minimum contacts" test for a court's jurisdiction in a case. select one: a. brown v. board of education of topeka, 347 u. s. 483 (1954). b. international shoe co. v. washington, 326 u. s. 310 (1945) c. haynes v. gore, 531 u. s. 98 (2000). d. international shoe co. v. washington, 14 u. s. code 336.
Answers: 1

Computers and Technology, 24.06.2019 01:00, kkruvc
Mastercard managers are motivated to increase (1) the number of individuals who have and use a mastercard credit card, (2) the number of banks and other clents who issue mastercards to customers and/or employees, and (3) the number of locations that accept mastercard payments. discuss how mastercard could use its data warehouse to it expand each of these customer bases.
Answers: 3

Computers and Technology, 24.06.2019 23:30, clairajogriggsk
What is the opening page of a website called? a. web page b. landing page c. homepage d. opening page
Answers: 1
You know the right answer?
Here is the starting code for your assignment. As you can see, so far there is a function to create...
Questions in other subjects:




Mathematics, 30.10.2019 04:31




