
Computers and Technology, 02.08.2021 19:50 jackolantern1437
Here is the starting code for your assignment. As you can see, so far there is a function to create two random numbers between 1 and 25 and a function to add them together. Your assignment is to create three more functions, add the prototypes correctly, call them from main correctly and output the results. The three functions should be subtractNumbers, multiplyNumbers, and divideNumbers. Remember that division won't always yield an integer so be sure to take care of that issue within the function (don't change the variable declarations).
Don't change the code that I've given you.
Follow the same pattern.
Don't use tools that we haven't studied.
// This program adds, subtracts, multiplies, and divides two random numbers
#include
#include // For rand and srand
#include // For the time function
using namespace std;
// Function declarations
int chooseNumber();
int addNumbers(int, int);
const int MIN_VALUE = 1; // Minimum value
const int MAX_VALUE = 25; // Maximum value
unsigned seed = time(0);
int main()
{
// Seed the random number generator.
srand(seed);
int firstNumber = chooseNumber();
int secondNumber = chooseNumber();
int sum = addNumbers(firstNumber, secondNumber);
cout << "The first number is " << firstNumber << endl;
cout << "The second number is " << secondNumber << endl;
cout << "The sum is " << sum;
return 0;
}
int chooseNumber()
{
// Variable
int number; // To hold the value of the number
number = (rand() % (MAX_VALUE - MIN_VALUE + 1)) + MIN_VALUE;
return number;
}
int addNumbers (int number1, int number2)
{
int number = number1 +number2;
return number;
}
Notice that number is used as a variable in more than one function. This works because number is a variable.

Answers: 1

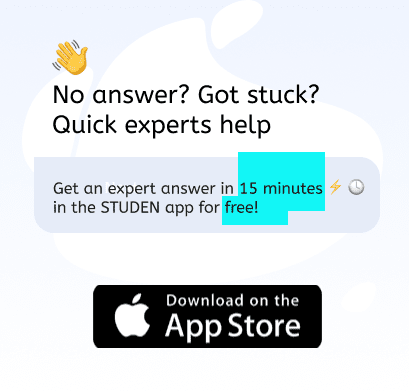
Other questions on the subject: Computers and Technology

Computers and Technology, 21.06.2019 22:00, richellemjordan
Draw the hierarchy chart and design the logic for a program that calculates service charges for hazel's housecleaning service. the program contains housekeeping, detail loop, and end-of-job modules. the main program declares any needed global variables and constants and calls the other modules. the housekeeping module displays a prompt for and accepts a customer's last name. while the user does not enter for the name, the detail loop accepts the number of bathrooms and the number of other rooms to be cleaned. the service charge is computed as $40 plus $15 for each bathroom and $10 for each of the other rooms. the detail loop also displays the service charge and then prompts the user for the next customer's name. the end-of-job module, which executes after the user enters the sentinel value for the name, displays a message that indicates the program is complete.
Answers: 2


Computers and Technology, 23.06.2019 00:30, devenybates
Which one of the following is the most accurate definition of technology? a electronic tools that improve functionality b electronic tools that provide entertainment or practical value c any type of tool that serves a practical function d any type of tool that enhances communication
Answers: 1

Computers and Technology, 23.06.2019 03:00, Julianhooks
State 7 common key's for every keyboard
Answers: 1
You know the right answer?
Here is the starting code for your assignment. As you can see, so far there is a function to create...
Questions in other subjects:

Mathematics, 10.12.2020 23:00



Social Studies, 10.12.2020 23:00





Mathematics, 10.12.2020 23:00

Mathematics, 10.12.2020 23:00