
Computers and Technology, 07.06.2021 09:50 christianmcafee
Question: 4
Consider the following correct implementation of the selectionsort algorithm:
public static ArrayList selectionSort(ArrayList arr){ int currentMinIndex; //Line 3 int counter = 0; for (int i = 0; i < arr. size() - 1; i++) //Line 5 { currentMinIndex = i; for (int j = i + 1; j < arr. size(); j++) { if(arr. get(j) < arr. get(currentMinIndex)) //Line 10 { currentMinIndex = j; } } if (i != currentMinIndex) //Line 16 { int temp = arr. get(currentMinIndex); arr. set(currentMinIndex, arr. get(i)); //Line 19 arr. set(i, temp); } } return arr;}
What would be the most effective way to alter this selectionsort algorithm so that an ArrayList would be sorted from greatestto smallest value?
Change Line 3 to int currentMaxIndex;
Change Line 5 to for(int i= arr. size() -1; i > 0; i --)
Change Line 10 to if(arr. get(j) >arr. get(currentMinIndex))
Change Line 16 to if (i > currentMinIndex)
Change Line 19 to arr. set(arr. get(i), currentMinIndex);
Question: 11
Consider the following correct implementation of the insertionsort algorithm:
public static int[] insertionSort(int[] arr){ for (int i = 1; i < arr. length; i++) { int curNumber = arr[i]; int curIndex = i-1; while ( curIndex >= 0 && arr[curIndex] > curNumber) { arr[curIndex+1] = arr[curIndex]; curIndex--; //Line 13 } arr[curIndex + 1] = curNumber; } return arr;}
The following declaration and method call are made in anothermethod in the same class as insertionSort:
int[] nums = {6, 5, 4, 3, 2, 1};list = insertionSort(nums);
How many times is the statement on Line 13 executed as a resultof the call to insertionSort?
5
30
15
16
6
Question: 13
Consider the following correct implementation of the selectionsort algorithm:
1. public static ArrayList selectionSort(ArrayList arr)2. {3. int currentMinIndex;4. int counter = 0;5. for (int i = 0; i < arr. size() - 1; i++)6. {7. currentMinIndex = i;8. for (int j = i + 1; j < arr. size(); j++)9. {10. if(arr. get(j) < arr. get(currentMinIndex))11. {12. currentMinIndex = j;13. }14. }15. if (i != currentMinIndex)16. {17. int temp = arr. get(currentMinIndex);18. arr. set(currentMinIndex, arr. get(i));19. arr. set(i, temp); //Line 1920. }21. }22. return arr;23. }
Given an ArrayList initialized with the values [6, 5, 4, 3, 2,1], how many times does Line 19 execute when the ArrayList issorted using the selection sort algorithm?
6
3
5
30
4
Question: 17
A website organizes its list of contributors in alphabeticalorder by last name. The website’s new manager would prefer thecontributor list to be ordered in reverse alphabetical orderinstead. Which classic algorithm would be best suited to completethis task?
Linear/Sequential Search
Selection Sort
Insertion Sort
None of these algorithms are viable options.
Question: 18
The following method is a search method intended to return theindex at which a String value occurs within an ArrayList:
public int search(ArrayList list, String target) //Line 1{ int counter = 0; while(counter < list. size()) //Line 4 { if(list. get(counter).equals(target)) { return list. get(counter); //Line 8 } counter++; //Line 10 } return -1; //Line 12}
However, there is currently an error preventing the method towork as intended. Which of the following Lines needs to be modifiedin order for the method to work as intended?
Line 1 - The method should be returning a String value, not anint.
Line 4 - The loop should go to < list. size() - 1 so as toavoid an .
Line 8 - The return should be the counter, notlist. get(counter).
Line 10 - As written, the counter will skip every othervalue.
Line 12 - The return value should be a String, not an int.
Question: 19
A company organizes all of its client database in order of theamount of money that the account is worth. When a new account isadded, it is placed in the correct order in the database based onthe worth of the account. Which classic algorithm would be bestsuited to perform this function?
Linear/Sequential Search
Insertion Sort
Selection Sort
None of these are viable options

Answers: 3

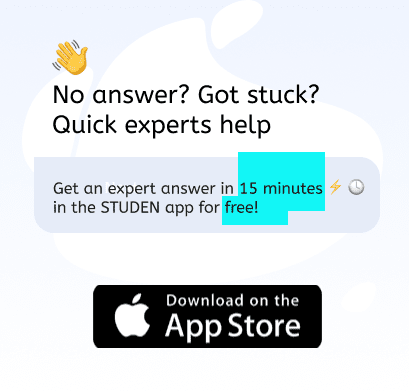
Other questions on the subject: Computers and Technology


Computers and Technology, 22.06.2019 18:30, leannhb3162
Which of these options are the correct sequence of actions for content to be copied and pasted? select content, click the copy button, click the paste button, and move the insertion point to where the content needs to be inserted. click the copy button, select the content, move the insertion point to where the content needs to be inserted, and click the paste button. select the content, click the copy button, move the insertion point to where the content needs to be inserted, and click the paste button. select the content, move the insertion point to where the content needs to be inserted, click the copy button, and click the paste button.
Answers: 3


Computers and Technology, 24.06.2019 10:20, silviamgarcia
Write a program that keeps asking the user for new values to be added to a list until the user enters 'exit' ('exit' should not be added to the list). these values entered by the user are added to a list we call 'initial_list'. then write a function that takes this initial_list as input and returns another list with 3 copies of every value in the initial_list. finally, inside print out all of the values in the new list. for example: input: enter value to be added to list: a enter value to be added to list: b enter value to be added to list: c enter value to be added to list: exit output: a b c a b c a b c note how 'exit' is not added to the list. also, your program needs to be able to handle any variation of 'exit' such as 'exit', 'exit' etc. and treat them all as 'exit'.
Answers: 2
You know the right answer?
Question: 4
Consider the following correct implementation of the selectionsort algorithm:
Questions in other subjects:

Mathematics, 10.06.2020 21:57

Mathematics, 10.06.2020 21:57






History, 10.06.2020 21:57

Mathematics, 10.06.2020 21:57