
Computers and Technology, 02.05.2021 04:00 sara12340
Write a MIPS assembly language program that prompts for a user to enter a series of floating point numbers and calls read_float to read in numbers and store them in an array only if the same number is not stored in the array yet. Then the program should display the array content on the console window.
Consult the green sheet and the chapter 3 for assembly instructions for floating point numbers. Here is one instruction that you might use:
c. eq. s $f2, $f4
bc1t Label1
Here if the value in the register $f2 is equals to the value in $f4, it jumps to the Label1. If it should jump when the value in the register $f2 is NOT equals to the value in $f4, then it should be:
c. eq. s $f2, $f4
bc1f Label1
To load a single precision floating point number (instead of lw for an integer), you might use:
l. s $f12, 0($t0)
To store a single precision floating point number (instead of sw for an integer), you might use:
s. s $f12, 0($t0)
To assign a constant floating point number (instead of li for an integer), you might use:
li. s $f12, 123.45
To copy a floating point number from one register to another (instead of move for an integer), you might use:
mov. s $f10, $f12
The following shows the syscall numbers needed for this assignment.
System Call System Call System Call
Number Operation Description
2 print_float $v0 = 2, $f12 = float number to be printed
4 print_string $v0 = 4, $a0 = address of beginning of ASCIIZ string
6 read_float $v0 = 6; user types a float number at keyboard; value is store in $f0
8 read_string $v0 = 8; user types string at keybd; addr of beginning of string is store in $a0; len in $a1
C program will ask a user to enter numbers and store them in an array
only if the same number is not in the array yet.
Then it prints out the result array content.
You need to write MIPS assembly code based on the following C code.
void main( )
{
int arraysize = 10;
float array[arraysize];
int i, j, alreadyStored;
float num;
i = 0;
while (i < arraysize)
{
printf("Enter a number:\n");
//read an integer from a user input and store it in num1
scanf("%f", &num);
//check if the number is already stored in the array
alreadyStored = 0;
for (j = 0; j < i; j++)
{
if (array[j] == num)
{
alreadyStored = 1;
}
}
//Only if the same number is not in the array yet
if (alreadyStored == 0)
{
array[i] = num;
i++;
}
}
printf("The array contains the following:\n");
i = 0;
while (i < arraysize)
{
printf("%f\n", array[i]);
i++;
}
return;
}
Here are sample outputs (user input is in bold): -- note that you might get some rounding errors
Enter a number:
3
Enter a number:
54.4
Enter a number:
2
Enter a number:
5
Enter a number:
2
Enter a number:
-4
Enter a number:
5
Enter a number:
76
Enter a number:
-23
Enter a number:
43.53
Enter a number:
-43.53
Enter a number:
43.53
Enter a number:
65.43
The array contains the following:
3.00000000
54.40000153
2.00000000
5.00000000
-4.00000000
76.00000000
-23.00000000
43.52999878
-43.52999878
65.43000031

Answers: 1

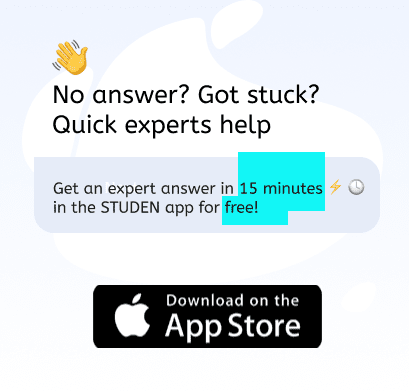
Other questions on the subject: Computers and Technology

Computers and Technology, 23.06.2019 07:00, Dvrsug8598
You need a quick answer from a coworker. the most effective way to reach your coworker is through a. cloud server b. instant message c. teleconference d. telepresence
Answers: 1

Computers and Technology, 23.06.2019 16:00, CalCDanG
What is the biggest difference between section breaks and regular page breaks? section breaks are more difficult to add than page breaks. section breaks make it easier for you to view the document as an outline. section breaks allow you to have areas of the document with different formatting. section breaks are smaller than regular page breaks.
Answers: 2

Computers and Technology, 23.06.2019 23:00, minosmora01
How do you know if the website is secure if you make a purchase
Answers: 2
You know the right answer?
Write a MIPS assembly language program that prompts for a user to enter a series of floating point n...
Questions in other subjects:

Mathematics, 02.06.2020 02:59





Chemistry, 02.06.2020 02:59


Biology, 02.06.2020 02:59

Mathematics, 02.06.2020 02:59

Mathematics, 02.06.2020 02:59