
Computers and Technology, 23.04.2021 15:50 rakanmadi87
Consider the following problem: Input: Two sorted arrays, A, B. A and B together contain n integers. Output: The union and the intersection of A and B. For example, if A and B are: {0, 0, 0, 1, 2, 3, 97, 98} {0, 1, 2, 3, 4, 4, 10, 98, 100, 100} The union of A and B should be {0, 1, 2, 3, 4, 10, 97, 98, 100}. The intersection of A and B should be {0, 1, 2, 3, 98}. There should be NO duplicates in the union and intersection. Give an in-place algorithm that solves this problem in O(n) time. (i) describe the idea behind your algorithm in English (ii) Complete the method of union() and intersection(); (iii) analyze its running time. Regarding requirement (iii): Unless otherwise specified, show the steps of your analysis and present your result using big-o. public class Problemi { public static void intersection(int[] si, int[] s2) { // complete the intersection() method to output // elements that occur in both si and s2 // feel free to change method type and parameters // Full credit will awarded to algorithms O(n) and in-place } { public static void union(int[] si, int[] s2) // complete the union() method to output // the union s1 and s2 // feel free to change method type and parameters // Full credit will awarded to algorithms O(n) and in-place } public static void main(String[] args) { // TODO Auto-generated method stub // Test your intersection() method here int[] testarray1 = {0, 0, 0, 1, 2, 3, 97, 98}; int[] testarray2 = {0, 1, 2, 3, 4, 4, 10, 98, 100, 100); System. out. println("intersection of testarray1 and testarray2: "); intersection(testarray1, testarray2); //should output 0, 1, 2, 3, 98 System. out. println("union of testarray1 and testarray2: "); union(testarrayı, testarray2); // should output 0, 1, 2, 3, 4, 10, 97, 98, 100 } }

Answers: 3

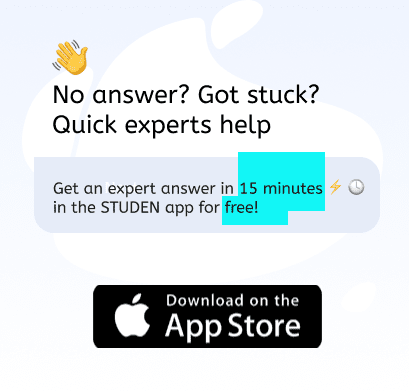
Other questions on the subject: Computers and Technology

Computers and Technology, 22.06.2019 03:10, brylove603
Write a program that begins by reading in a series of positive integers on a single line of input and then computes and prints the product of those integers. integers are accepted and multiplied until the user enters an integer less than 1. this final number is not part of the product. then, the program prints the product. if the first entered number is negative or 0, the program must print “bad input.” and terminate immediately. next, the program determines and prints the prime factorization of the product, listing the factors in increasing order. if a prime number is not a factor of the product, then it must not appear in the factorization. sample runs are given below. note that if the power of a prime is 1, then that 1 must appear in t
Answers: 3

Computers and Technology, 22.06.2019 10:20, vuqepete4528
Shown below is the start of a coding region within the fist exon of a gene. 5'--3' 3'--5' how many cas9 pam sequences are present?
Answers: 1

Computers and Technology, 22.06.2019 17:30, kameronstebbins
Which tab should you open to find the option for adding a header?
Answers: 1

Computers and Technology, 24.06.2019 04:30, littledudefromacross
Write and test a python program to find and print the largest number in a set of real (floating point) numbers. the program should first read a single positive integer number from the user, which will be how many numbers to read and search through. after reading in all of the numbers, the largest of the numbers input (not considering the count input) should be printed.
Answers: 1
You know the right answer?
Consider the following problem: Input: Two sorted arrays, A, B. A and B together contain n integers....
Questions in other subjects:







History, 13.02.2020 20:52


Geography, 13.02.2020 20:52