
Computers and Technology, 18.04.2021 01:00 leslieperez67
Create an application in C# named CarDemo that declares at least two Car objects and demonstrates how they can be incremented using an overloaded ++ operator.
Create a Car class that contains the following properties:
Model - The car model (as a string)
Mpg The car's miles per gallon (as a double)
Include two overloaded constructors. One accepts parameters for the model and miles per gallon; the other accepts a model and sets the miles per gallon to 20.
Overload the ++ operator that increases the miles per gallon value by 1. The CarDemo application creates at least one Car using each constructor and displays the Car values both before and after incrementation.
this is what i have:
using static System. Console;
class CarDemo
{
static void Main()
{
Car c1 = new Car("Camero", 25);
Car c2 = new Car("Mustang");
Display("Camero at beginning", c1);
++c1;
Display("Camero after prefix increment", c1);
Display("Mustang at beginning", c2);
++c2;
Display("Mustang after prefix increment", c2);
}
public static void Display(string message, Car)
{
WriteLine(Mpg);
}
class Car
{
private string Model
{
get
{
return model;
}
set
{
model = value;
}
}
private double Mpg
{
get
{
return mpg;
}
set
{
mpg = value;
}
}
public Car(string model, double mpg)
{
Model = model;
Mpg = mpg;
}
public Car(string model)
{
Model = model;
Mpg = 20;
}
public static Car operator++(Car c)
{
++c. Mpg;
return c;
}
}
}
what am i doing wrong?

Answers: 1

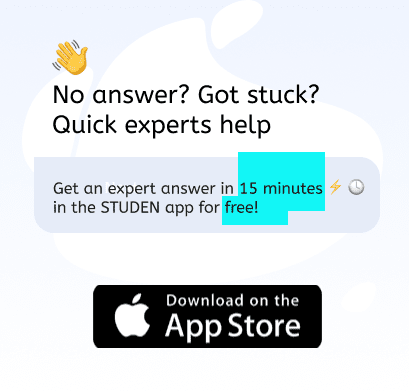
Other questions on the subject: Computers and Technology

Computers and Technology, 22.06.2019 21:00, bryanatwin1536
Describir textbook icon_person mira los dibujos y describe lo que está pasando. usa los verbos de la lista.
Answers: 1

Computers and Technology, 23.06.2019 02:00, mayapril813
Consider the following function main: int main() { int alpha[20]; int beta[20]; int matrix[10][4]; . . } a. write the definition of the function inputarray that prompts the user to input 20 numbers and stores the numbers into alpha. b. write the definition of the function doublearray that initializes the elements of beta to two times the corresponding elements in alpha. make sure that you prevent the function from modifying the elements of alpha. c. write the definition of the function copyalphabeta that stores alpha into the first five rows of matrix and beta into the last five rows of matrix. make sure that you prevent the function from modifying the elements of alpha and beta. d. write the definition of the function printarray that prints any onedimensional array of type int. print 15 elements per line. e. write a c11 program that tests the function main and the functions discussed in parts a through d. (add additional functions, such as printing a two-dimensional array, as needed.)
Answers: 3


Computers and Technology, 25.06.2019 00:00, jessica2138
He computer component that disperses heat from the microprocessor to the cooling fan is a cooler thermometer heat sink
Answers: 1
You know the right answer?
Create an application in C# named CarDemo that declares at least two Car objects and demonstrates ho...
Questions in other subjects:

Mathematics, 19.04.2021 16:10







