
Computers and Technology, 13.04.2021 02:30 amcdonald009
This program maintains a linked list of students, where each student contains a name (one-word only) and a GPA. The program operates as follows:
Prompts the user for names and GPAs, building the list with calls to add, printing the list after each add
Prompts the user for a name, and then prints the GPA of that student (-1 if the name is not in the list)
You must complete the following:
Write the function getGPA that returns the gpa of the name passed to it, or -1.0 if the name doesn’t exist.
Re-write add so that all the students will be ordered by descending GPA. For those with the same GPA, they will be ordered ascending alphabetically.
Do not add a name if it already exists in the list and print the error message: "Student named %s exists.\n"
It is recommended to write a helper function compareStudents that compares two students and returns -1, 1 or 0, depending on the positions of the two students in the sorted linked list.
Two sample inputs for the program are shown below:
Fred 3.5
Barney 2.4
Betty 3.9
Wilma 3.5
xxx 0
Fred
Betty
xxx
Betty 3.5
Fred 2.5
Fred 2.9
Wilma 2.9
Wilma 3.0
xxx 0
Pebbles
xxx
Code :
#include
#include
#include
typedef struct _student {
char *name;
double gpa;
struct _student *next;
} Student;
Student *createStudent(char name[], double gpa, Student *next) {
Student *pNew = malloc(sizeof(Student));
pNew->name = malloc(strlen(name) + 1);
strcpy(pNew->name, name);
pNew->gpa = gpa;
pNew->next = next;
return pNew;
}
int compareStudents(Student *pNew, Student *pExisting) {
//code here
return -1;
}
Student *add(Student *head, char name[], double gpa) {
//code here
return createStudent(name, gpa, head);
}
void print(char prefix[], Student *head) {
printf("%s", prefix);
for (Student *ptr = head; ptr != NULL; ptr = ptr->next)
printf("[%s-%g] ", ptr->name, ptr->gpa);
printf("\n");
}
double getGPA(Student *head, char name[]) {
//code here
return -1.0;
}
int main(void) {
char name[100];
double gpa;
Student *head = NULL;
while (1) { // build the list
printf("\nEnter a name (one word) and gpa, or xxx and 0 to exit: ");
scanf("%s%lf", name, &gpa);
if (strcmp(name, "xxx") == 0) break;
head = add(head, name, gpa);
print("\n\tNow the list is: ", head);
}
while (1) { // perform search
printf("\nEnter a name to look up the gpa or xxx to exit: ");
scanf("%s", name);
if (strcmp(name, "xxx") == 0) break;
gpa = getGPA(head, name);
if (gpa < 0)
printf("\n\t%s does not exist\n", name);
else
printf("\n\t%s has a GPA of %g\n", name, gpa);
}
return 0;
}

Answers: 2

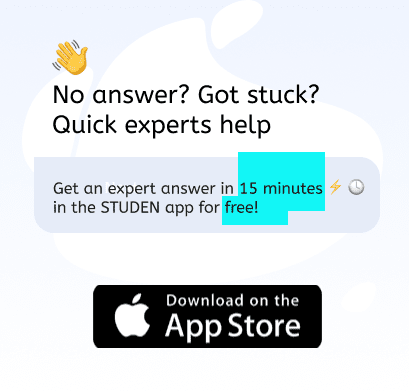
Other questions on the subject: Computers and Technology

Computers and Technology, 22.06.2019 08:10, josued123321
Alook-up table used to convert pixel values to output values on a monitor. essentially, all pixels with a value of 190 or above are shown as white (i. e. 255), and all values with a value of 63 or less are shown as black (i. e. 0). in between the pixels are scaled so that a pixel with a value p is converted to a pixel of value 2/127 −+3969). if a pixel has a value of 170 originally, what value will be used to display the pixel on the monitor? if a value of 110 is used to display the pixel on the monitor, what was the original value of the pixel?
Answers: 1

Computers and Technology, 22.06.2019 14:10, normarismendoza
Dean wants a quick way to look up staff members by their staff id. in cell q3, nest the existing vlookup function in an iferror function. if the vlookup function returns an error result, the text “invalid staff id” should be displayed by the formula. (hint: you can test that this formula is working by changing the value in cell q2 to 0, but remember to set the value of cell q2 back to 1036 when the testing is complete.)
Answers: 3

Computers and Technology, 22.06.2019 22:30, reinasuarez964
One of your customers wants you to build a personal server that he can use in his home. one of his concerns is making sure he has at least one backup of their data stored on the server in the event that a disk fails. you have decided to back up his data using raid. since this server is for personal use only, the customer wants to keep costs down. therefore, he would like to keep the number of drives to a minimum. which of the following raid systems would best meet the customer's specifications? a. raid 0 b. raid 1 c. raid 5 d. raid 10
Answers: 3
You know the right answer?
This program maintains a linked list of students, where each student contains a name (one-word only)...
Questions in other subjects:


Mathematics, 06.11.2020 01:00

English, 06.11.2020 01:00

English, 06.11.2020 01:00

Mathematics, 06.11.2020 01:00

Mathematics, 06.11.2020 01:00


Mathematics, 06.11.2020 01:00
