
Computers and Technology, 05.04.2021 23:10 sarbjit879
Given main() and the Instrument class, define a derived class, StringInstrument, for string instruments.
Ex. If the input is:
Drums
Zildjian
2015
2500
Guitar
Gibson
2002
1200
6
19
the output is:
Instrument Information:
Name: Drums
Manufacturer: Zildjian
Year built: 2015
Cost: 2500
Instrument Information:
Name: Guitar
Manufacturer: Gibson
Year built: 2002
Cost: 1200
Number of strings: 6
Number of frets: 19
//main. cpp
#include "StringInstrument. h"
int main() {
Instrument myInstrument;
StringInstrument myStringInstrument;
string instrumentName, manufacturerName, stringInstrumentName, stringManufacturer, yearBuilt,
cost, stringYearBuilt, stringCost, numStrings, numFrets;
getline(cin, instrumentName);
getline(cin, manufacturerName);
getline(cin, yearBuilt);
getline(cin, cost);
getline(cin, stringInstrumentName);
getline(cin, stringManufacturer);
getline(cin, stringYearBuilt);
getline(cin, stringCost);
getline(cin, numStrings);
getline(cin, numFrets);
myInstrument. SetName(instrumentName);
myInstrument. SetManufacturer(manufacturerName);< br />
myInstrument. SetYearBuilt(yearBuilt);
myInstrument. SetCost(cost);
myInstrument. PrintInfo();
myStringInstrument. SetName(stringInstrumentName);
myStringInstrument. SetManufacturer(stringManufacturer) ;
myStringInstrument. SetYearBuilt(stringYearBuilt);
myStringInstrument. SetCost(stringCost);
myStringInstrument. SetNumOfStrings(numStrings);
myStringInstrument. SetNumOfFrets(numFrets);
myStringInstrument. PrintInfo();
cout << " Number of strings: " << myStringInstrument. GetNumOfStrings() << endl;
cout << " Number of frets: " << myStringInstrument. GetNumOfFrets() << endl;
}
//Instrument. h
#ifndef INSTRUMENTH
#define INSTRUMENTH
#include
#include
using namespace std;
class Instrument {
protected:
string instrumentName;
string instrumentManufacturer;
string yearBuilt;
string cost;
public:
void SetName(string userName);
string GetName();
void SetManufacturer(string userManufacturer);
string GetManufacturer();
void SetYearBuilt(string userYearBuilt);
string GetYearBuilt();
void SetCost(string userCost);
string GetCost();
void PrintInfo();
};
#endif
//Instrument. cpp
#include "Instrument. h"
void Instrument::SetName(string userName) {
instrumentName = userName;
}
string Instrument::GetName() {
return instrumentName;
}
void Instrument::SetManufacturer(string userManufacturer) {
instrumentManufacturer = userManufacturer;
}
string Instrument::GetManufacturer() {
return instrumentManufacturer;
}
void Instrument::SetYearBuilt(string userYearBuilt) {
yearBuilt = userYearBuilt;
}
string Instrument::GetYearBuilt() {
return yearBuilt;
}
void Instrument::SetCost(string userCost) {
cost = userCost;
}
string Instrument::GetCost() {
return cost;
}
void Instrument::PrintInfo() {
cout << "Instrument Information: " << endl;
cout << " Name: " << instrumentName << endl;
cout << " Manufacturer: " << instrumentManufacturer << endl;
cout << " Year built: " << yearBuilt << endl;
cout << " Cost: " << cost << endl;
}
//StringInstrument. h
#ifndef STR_INSTRUMENTH
#define STR_INSTRUMENTH
#include "Instrument. h"
class StringInstrument : public Instrument {
// TODO: Declare private data members: numStrings, numFrets
// TODO: Declare mutator functions -
// SetNumOfStrings(), SetNumOfFrets()
// TODO: Declare accessor functions -
// GetNumOfStrings(), GetNumOfFrets()
};
#endif
//StringInstrument. cpp
#include "StringInstrument. h"
// TODO: Define mutator functions -
// SetNumOfStrings(), SetNumOfFrets()
// TODO: Define accessor functions -
// GetNumOfStrings(), GetNumOfFrets()

Answers: 2

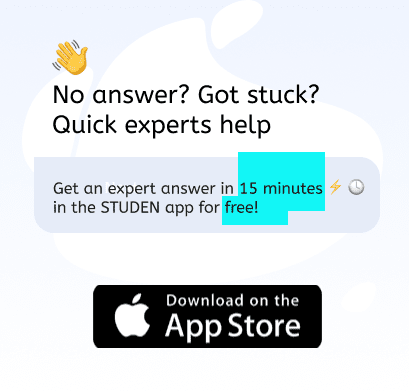
Other questions on the subject: Computers and Technology

Computers and Technology, 22.06.2019 10:30, darrengresham999
Choose the best explanation for the following statement communication is symbolic
Answers: 3

Computers and Technology, 22.06.2019 14:20, babyrocks7300
Consider a byte-addressable computer with 16mb of main memory, a cache capable of storing a total of 64kb of data and block size of 32 bytes. (a) how many bits in the memory address? (b) how many blocks are in the cache? (c) specify the format of the memory address, including names and sizes, when the cache is: 1. direct-mapped 2. 4-way set associative 3. fully associative
Answers: 2

Computers and Technology, 22.06.2019 22:30, josephmelichar777
Write a full class definition for a class named player , and containing the following members: a data member name of type string .a data member score of type int .a member function called setname that accepts a parameter and assigns it to name . the function returns no value. a member function called setscore that accepts a parameter and assigns it to score . the function returns no value. a member function called getname that accepts no parameters and returns the value of name .a member function called getscore that accepts no parameters and returns the value of score .this is what i have, aparently this is wrong: class player{private: string name; int score; public: void player: : setname (string n){name =n; }void player: : setscore (int s){score = s; }string player: : getname (){return name; }int player: : getscore (){return score; }};
Answers: 2
You know the right answer?
Given main() and the Instrument class, define a derived class, StringInstrument, for string instrume...
Questions in other subjects:

English, 20.01.2021 14:50



Medicine, 20.01.2021 14:50


Mathematics, 20.01.2021 14:50

Spanish, 20.01.2021 14:50

Advanced Placement (AP), 20.01.2021 14:50

Social Studies, 20.01.2021 14:50

Social Studies, 20.01.2021 14:50