
Computers and Technology, 02.04.2021 21:50 RainbowPieWasHere
Coding exercise on Python dictionaries. Look at the DNA translation code (with the included dictionary for the codons to amino acids),
STANDARD_GENETIC_CODE = { 'UUU':'Phe', 'UUC':'Phe', 'UCU':'Ser', 'UCC':'Ser', 'UAU':'Tyr', 'UAC':'Tyr', 'UGU':'Cys', 'UGC':'Cys', 'UUA':'Leu', 'UCA':'Ser', 'UAA':None, 'UGA':None, 'UUG':'Leu', 'UCG':'Ser', 'UAG':None, 'UGG':'Trp', 'CUU':'Leu', 'CUC':'Leu', 'CCU':'Pro', 'CCC':'Pro', 'CAU':'His', 'CAC':'His', 'CGU':'Arg', 'CGC':'Arg', 'CUA':'Leu', 'CUG':'Leu', 'CCA':'Pro', 'CCG':'Pro', 'CAA':'Gln', 'CAG':'Gln', 'CGA':'Arg', 'CGG':'Arg', 'AUU':'Ile', 'AUC':'Ile', 'ACU':'Thr', 'ACC':'Thr', 'AAU':'Asn', 'AAC':'Asn', 'AGU':'Ser', 'AGC':'Ser', 'AUA':'Ile', 'ACA':'Thr', 'AAA':'Lys', 'AGA':'Arg', 'AUG':'Met', 'ACG':'Thr', 'AAG':'Lys', 'AGG':'Arg', 'GUU':'Val', 'GUC':'Val', 'GCU':'Ala', 'GCC':'Ala', 'GAU':'Asp', 'GAC':'Asp', 'GGU':'Gly', 'GGC':'Gly', 'GUA':'Val', 'GUG':'Val', 'GCA':'Ala', 'GCG':'Ala', 'GAA':'Glu', 'GAG':'Glu', 'GGA':'Gly', 'GGG':'Gly'}
def proteinTranslation(seq, geneticCode): """ This function translates a nucleic acid sequence into a protein sequence, until the end or until it comes across a stop codon """ seq = seq. replace('T','U') # Make sure we have RNA sequence proteinSeq = [] i = 0 while i+2 < len(seq): codon = seq[i:i+3] aminoAcid = geneticCode[codon] if aminoAcid is None: # Found stop codon break proteinSeq. append(aminoAcid) i += 3
Give a short verbal description of what the code does on each line. Then start typing the code out on the online Python interpreter, but use an alternative dictionary with key-values for codons to amino acid called NON_STANDARD_GENETIC_CODE. Just type the first 20 key-value pairs or so from the STANDARD_GENETIC_CODE dictionary shown in the book. Now what you need to do for this exercise is to modify the "def proteintranslation" function, so that it has an "if"statement before the line where it pulls the codons from the dictionary. With this modification, our code will first check if that codon exists in the dictionary, and if not it sets the codon to a default codon='X', else it sets the default codon = seq[i:i+3]. You need to have the code working by writing out the def function, and demonstrating that you can call the function with a DNA sequence, and print out the translation of the DNA string. Try it with a few different DNA strings, and see how many X's you get.

Answers: 2

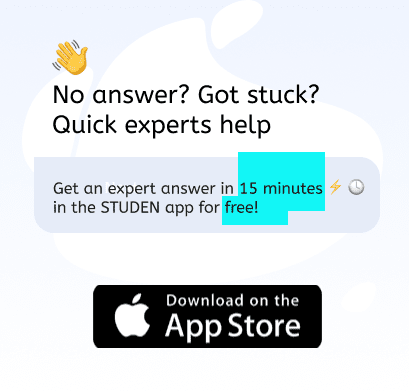
Other questions on the subject: Computers and Technology

Computers and Technology, 23.06.2019 01:10, kristofwr3444
Are special combinations of keys that tell a computer to perform a command. keypads multi-keys combinations shortcuts
Answers: 1


Computers and Technology, 24.06.2019 16:00, dareaalcaam111
What is a dashed line showing where a worksheet will be divided between pages when it prints? a freeze pane a split box a page break a print title
Answers: 1

Computers and Technology, 25.06.2019 05:10, mathbrain58
Write a program that asks for 'name' from the user and then asks for a number and stores the two in a dictionary (called 'the_dict') as key-value pair. the program then asks if the user wants to enter more data (more data (y/n)? ) and depending on user choice, either asks for another name-number pair or exits and stores the dictionary key, values in a list of tuples and prints the list. note: ignore the case where the name is already in the dictionary. example: name: pranshu number: 517-244-2426 more data (y/n)? y name: rich number: 517-842-5425 more data (y/n)? y name: alireza number: 517-432-5224 more data (y/n)? n [('alireza', '517-432-5224'), ('pranshu', '517-244-2426'), ('rich', '517-842-5425')]
Answers: 3
You know the right answer?
Coding exercise on Python dictionaries. Look at the DNA translation code (with the included dictiona...
Questions in other subjects:



Mathematics, 30.08.2019 04:30



Mathematics, 30.08.2019 04:30

History, 30.08.2019 04:30


Mathematics, 30.08.2019 04:30