
Computers and Technology, 31.03.2021 03:20 pricilaxo9523
In this challenge, you will write a method called int countDiscountedItems() in the ShoppingCart class.
This method will use a loop to traverse the ArrayList of Items called order.
In the loop, you will test if each Item is a DiscountedItem by using the instanceof keyword ((object instanceof Class) returns true or false) similar to its use in the add(Item) method.
If it is a DiscountedItem, then you will count it.
At the end of the loop, the method will return the count.
Make sure you print out the number of discounted items in the main method or in printOrder(), so that you can test your method. Add more items to the order to test it.
Copy in your code for DiscountedItem below and then write a method called countDiscountedItems which traverses the polymorphic ArrayLists of Items. Use instanceOf to test items to see if they are a DiscountedItem.
import java. util.*;
/**
The ShoppingCart class has an ArrayList of Items.
You will write a new class DiscountedItem that extends Item.
This code is adapted https://practiceit. cs. washington. edu/problem/view/bjp4/chapter9/e10- DiscountBill
*/
public class Tester
{
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
cart. add(new Item("bread", 3.25));
cart. add(new Item("milk", 2.50));
//cart. add(new DiscountedItem("ice cream", 4.50, 1.50));
//cart. add(new DiscountedItem("apples", 1.35, 0.25));
cart. printOrder();
}
}
class DiscountedItem extends Item
{
// Copy your code from the last lesson's challenge here!
}
// Add a method called countDiscountedItems()
class ShoppingCart
{
private ArrayList order;
private double total;
private double internalDiscount;
public ShoppingCart()
{
order = new ArrayList();
total = 0.0;
internalDiscount = 0.0;
}
public void add(Item i) {
order. add(i);
total += i. getPrice();
if (i instanceof DiscountedItem)
internalDiscount += ((DiscountedItem) i).getDiscount();
}
/** printOrder() will call toString() to print */
public void printOrder() {
System. out. println(this);
}
public String toString() {
return discountToString();
}
public String discountToString() {
return orderToString() + "\nSub-total: " + valueToString(total) + "\nDiscount: " + valueToString(internalDiscount) + "\nTotal: " + valueToString(total - internalDiscount);
}
private String valueToString(double value) {
value = Math. rint(value * 100) / 100.0;
String result = "" + Math. abs(value);
if(result. indexOf(".") == result. length() - 2) {
result += "0";
}
result = "$" + result;
return result;
}
public String orderToString() {
String build = "\nOrder Items:\n";
for(int i = 0; i < order. size(); i++) {
build += " " + order. get(i);
if(i != order. size() - 1) {
build += "\n";
}
}
return build;
}
}
class Item {
private String name;
private double price;
public Item()
{
this. name = "";
this. price = 0.0;
}
public Item(String name, double price) {
this. name = name;
this. price = price;
}
public double getPrice() {
return price;
}
public String valueToString(double value) {
String result = "" + Math. abs(value);
if(result. indexOf(".") == result. length() - 2) {
result += "0";
}
result = "$" + result;
return result;
}
public String toString() {
return name + " " + valueToString(price);
}
}

Answers: 3

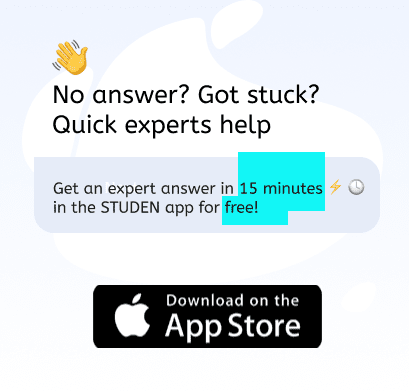
Other questions on the subject: Computers and Technology

Computers and Technology, 21.06.2019 18:10, ryan23allen
For each of the following claims, determine whether they are true or false. justify your determination (show your work). if the claim is false, state the correct asymptotic relationship as o, θ, or ω. unless otherwise specified, lg is log2.(a) (b) (c) (d) (e) (f) (g) (h) (i) (j)n+1 =22n =2n =1 =ln2 n =n2 +2n−4 =33n = 2n+1 =√n = 10100 =o(n4) o(2n)θ(2n+7 ) o(1/n)θ(lg2 n) ω(n2 )θ(9n ) θ(2n lg n )o(lg n) θ(1)
Answers: 1



Computers and Technology, 23.06.2019 16:00, lokaranjan5736
Write a grading program for a class with the following grading policies: a. there are two quizzes, each graded on the basis of 10 points. b. there is one midterm exam and one final exam, each graded on the basis of 100 points. c. the final exam counts for 50% of the grade, the midterm counts for 25%, and the two quizzes together count for a total of 25%. (do not forget to normalize the quiz scores. they should be converted to a percentage before they are averaged in.) any grade of 90 or more is an a, any grade of 80 or more (but less than 90) is a b, any grade of 70 or more (but less than 80) is a c, any grade of 60 or more (but less than 70) is a d, and any grade below 60 is an f. the program will read in the student’s scores and output the student’s record, which consists of two quiz and two exam scores as well as the student’s average numeric score for the entire course and final letter grade. define and use a structure for the student reco
Answers: 2
You know the right answer?
In this challenge, you will write a method called int countDiscountedItems() in the ShoppingCart cla...
Questions in other subjects:


English, 18.06.2021 20:30






Social Studies, 18.06.2021 20:30

Mathematics, 18.06.2021 20:30