
Computers and Technology, 19.03.2021 18:20 dreamdancekay
Design a stack class by importing the available java. util. Stack to have the following features:
push(x) -- push element x onto stack, where x is anywhere between Integer. MIN_VALUE and Integer. MAX_VALUE.
pop() -- remove the element on top of the stack.
top() -- get the top element.
getMax() -- retrieve the max element in the stack in constant time (i. e., O(1)).
Your code should have the following shape and form, all in one .java file. Note the styling and documentation API already included for the target class, MaxStack:
import java. util. Stack;
public class HomeworkAssignment1_1 {
public static void main(String[] args) {
// Your main() is not graded so you can
// have any implementation in this area
MaxStack obj = new MaxStack();
obj. push(12);
obj. push(1);
obj. push(-12);
obj. pop();
System. out. println(obj. top());
System. out. println(obj. getMax());
// etc.
}
}
/**
* The MaxStack program implements a Stack class with the following features:
* push(x) -- push element x onto stack
* pop() -- remove the element on top of the stack
* top() -- get the top element.
* getMax() -- retrieve the max element in the stack in constant time (i. e., O(1)
*/
class MaxStack {
// Initialize your data structure in constructor
// or here; choice is yours.
public MaxStack() { // YOUR CODE HERE }
public void push(int x) { // YOUR CODE HERE }
public void pop() { // YOUR CODE HERE }
public int top() { // YOUR CODE HERE }
public int getMax() { // YOUR CODE HERE }
}
EXAMPLES
MaxStack maxStack = new MaxStack();
maxStack. push(-2);
maxStack. push(0);
maxStack. push(-3);
maxStack. getMax(); // returns 0
maxStack. pop();
maxStack. top(); // returns 0
maxStack. getMax(); // returns 0
CONSTRAINTS AND ASSUMPTIONS
For this problem you are ONLY allowed to use Java's reference class Stack (Links to an external site.). Failure to do so will receive 5 points off.
MaxStack does not mean elements have to be ordered in increasing or decreasing values in the Stack.
HINTS
You solution should persist a global max value while maintaining the ability to transact on a java. util. Stack instance wrapped in your MaxStack class.
When implementing the MaxStack methods, you will have to invoke your Java stack instance's comparable methods (naming convention may be different).

Answers: 2

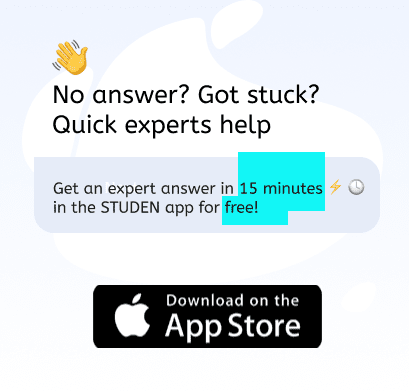
Other questions on the subject: Computers and Technology

Computers and Technology, 22.06.2019 08:50, najashohatee1234
Can online classes such as gradpoint track your ip location like if im taking a final and i give somebody else my account and they take the final for me will it show where they are taking the final from? and can this be countered with a vpn
Answers: 1

Computers and Technology, 24.06.2019 15:30, S917564
The idea that, for each pair of devices v and w, there’s a strict dichotomy between being “in range” or “out of range” is a simplified abstraction. more accurately, there’s a power decay function f (·) that specifies, for a pair of devices at distance δ, the signal strength f(δ) that they’ll be able to achieve on their wireless connection. (we’ll assume that f (δ) decreases with increasing δ.) we might want to build this into our notion of back-up sets as follows: among the k devices in the back-up set of v, there should be at least one that can be reached with very high signal strength, at least one other that can be reached with moderately high signal strength, and so forth. more concretely, we have values p1 ≥ p2 ≥ . . ≥ pk, so that if the back-up set for v consists of devices at distances d1≤d2≤≤dk, thenweshouldhavef(dj)≥pj foreachj. give an algorithm that determines whether it is possible to choose a back-up set for each device subject to this more detailed condition, still requiring that no device should appear in the back-up set of more than b other devices. again, the algorithm should output the back-up sets themselves, provided they can be found.\
Answers: 2

Computers and Technology, 24.06.2019 22:30, toricepeda82
What are the 4 basic items that are traded throughout the world?
Answers: 1
You know the right answer?
Design a stack class by importing the available java. util. Stack to have the following features:
p...
Questions in other subjects:








Social Studies, 19.02.2022 14:40
