Write a Queue class. The class is detailed as follows:
●__init__(self, capacity) → None
oComp...

Computers and Technology, 22.01.2021 17:20 french31
Write a Queue class. The class is detailed as follows:
●__init__(self, capacity) → None
oComplexity: O(1)
oValid Input: An integer in (0, ∞]. Use a default, valid capacity on invalid input.
oThis is the constructor for the class. You will need to store the following here:
▪The head pointer
▪The tail pointer
▪A variable maxSize
▪A variable currentSize
▪Any other instance variables you want.
●enqueue(self, ticket) → boolean
oComplexity: O(1)
oValid Input: An object of type: MealTicket. Return False on invalid input.
oThis is the enqueue method. It will add a meal ticket to the queue. It will then return True/False depending on if the enqueue was successful (Hint: Add a new ticket at the tail of the queue).
●dequeue(self) → MealTicket/ boolean
oComplexity: O(1)
oThis is the dequeue method. It will remove the ticket at the front of the queue andreturn it or False if the queue is empty. (Hint: Dequeue a ticket at the head of theQueue).
●front(self) → MealTicket/ boolean
oComplexity: O(1)
oThis method lets the user peak at the ticket at the front of the queue without deleting it. It will either return a meal ticket or false if the queue is empty.
●isEmpty(self) → boolean
oComplexity: O(1)
oThis method will return True/False depending on if the queue is empty or not.
●isFull(self) → boolean
oComplexity: O(1)
oThis method will return True/False depending on if the queue is full or not,
Your program must perform exactly as shown in the figure. Note 2: All methods in the queue class have a hard complexity upper bound of O(1). Your implementation must be in O(1). Note 3: For this assignment you must make and use your own linked list as the base data structure. Using lists, dictionaries or any other data structure will result in a 0 for the assignment

Answers: 1

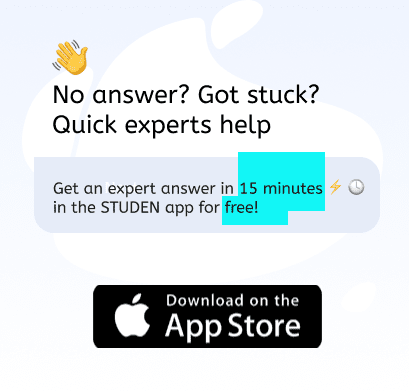
Other questions on the subject: Computers and Technology

Computers and Technology, 21.06.2019 16:30, ahmedislife
Select the correct answer. larry finds it easy to run legacy programs and applications in a virtualized environment. how does the virtualization provider make this possible? a. combines workloads of several underutilized servers to fewer machines b. installs and runs different versions of an operating system on the same computing device c. moves virtual machines from one server to another server at a different location d. streamlines and automates management tasks
Answers: 1

Computers and Technology, 22.06.2019 20:00, Jana1517
What is the worst-case complexity of the maxrepeats function? assume that the longest string in the names array is at most 25 characters wide (i. e., string comparison can be treated as o( class namecounter { private: int* counts; int nc; string* names; int nn; public: namecounter (int ncounts, int nnames); int maxrepeats() const; }; int namecounter: : maxrepeats () { int maxcount = 0; for (int i = 0; i < nc; ++i) { int count = 1; for (int j = i+1; j < nc; ++j) { if (names[i] == names[j]) ++count; } maxcount = max(count, maxcount); } return maxcount; }
Answers: 3


Computers and Technology, 23.06.2019 14:30, HarryPotter10
Open this link after reading about ana's situation. complete each sentence using the drop-downs. ana would need a minimum of ato work as a translator. according to job outlook information, the number of jobs for translators willin the future.
Answers: 3
You know the right answer?
Questions in other subjects:

Mathematics, 08.04.2020 20:03

Social Studies, 08.04.2020 20:03


Mathematics, 08.04.2020 20:03

Mathematics, 08.04.2020 20:03

Biology, 08.04.2020 20:03


Mathematics, 08.04.2020 20:03

English, 08.04.2020 20:03

Health, 08.04.2020 20:03