
Computers and Technology, 12.01.2021 18:00 abbbaayyyy1709
Given the Solid class, extend it with:
Pyramid
Cylinder
RectangularPrism
Sphere
Make sure to create the constructor and override the volume and surfaceArea methods.
Also extend RectangularPrism with Cube.
HINT: You can look up formulas for how to compute the volume and surface area of a certain type of shape online.
import java. lang. Math;
public class Pyramid extends Solid
{
// Code goes here
}
public class Cube extends RectangularPrism
{
// Code goes here
}
public class RectangularPrism extends Solid
{
// Code goes here
}
public class Solid
{
private String myName;
public Solid(String name)
{
myName = name;
}
public String getName()
{
return myName;
}
// This should be overriden in the subclass
public double volume()
{
return 0;
}
// This should be overriden in the subclass
public double surfaceArea()
{
return 0;
}
}
import java. lang. Math;
public class Sphere extends Solid
{
// Code goes here
}
import java. lang. Math;
public class Cylinder extends Solid
{
// Code goes here
}
public class SolidTester
{
public static void main(String[] args)
{
String name;
double volume;
double surfaceArea;
Pyramid pyramid = new Pyramid("My pyramid", 1, 3, 5);
name = pyramid. getName();
volume = round(pyramid. volume(), 2);
surfaceArea = round(pyramid. surfaceArea(), 2);
System. out. println("Pyramid '" + name + "' has volume: " + volume +
" and surface area: " + surfaceArea + ".");
Sphere sphere = new Sphere("My sphere", 4);
name = sphere. getName();
volume = round(sphere. volume(), 2);
surfaceArea = round(sphere. surfaceArea(), 2);
System. out. println("Sphere '" + name + "' has volume: " + volume +
" and surface area: " + surfaceArea + ".");
RectangularPrism rectangularPrism = new RectangularPrism("My rectangular prism",
5, 8, 3);
name = rectangularPrism. getName();
volume = round(rectangularPrism. volume(), 2);
surfaceArea = round(rectangularPrism. surfaceArea(), 2);
System. out. println("RectangularPrism '" + name + "' has volume: " +
volume + " and surface area: " + surfaceArea + ".");
Cylinder cylinder = new Cylinder("My cylinder", 4, 9);
name = cylinder. getName();
volume = round(cylinder. volume(), 2);
surfaceArea = round(cylinder. surfaceArea(), 2);
System. out. println("Cylinder '" + name + "' has volume: " + volume +
" and surface area: " + surfaceArea + ".");
Cube cube = new Cube("My cube", 4);
name = cube. getName();
volume = round(cube. volume(), 2);
surfaceArea = round(cube. surfaceArea(), 2);
System. out. println("Cube '" + name + "' has volume: " + volume +
" and surface area: " + surfaceArea + ".");
}
public static double round(double value, int places) {
if (places < 0) throw new IllegalArgumentException();
long factor = (long) Math. pow(10, places);
value = value * factor;
long tmp = Math. round(value);
return (double) tmp / factor;
}
}

Answers: 1

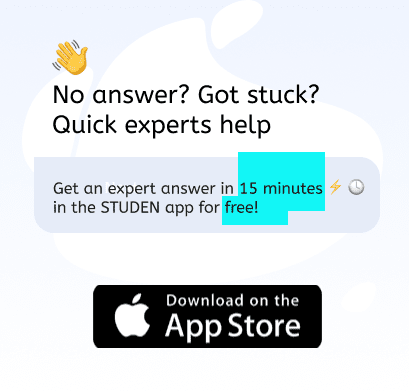
Other questions on the subject: Computers and Technology

Computers and Technology, 22.06.2019 12:30, kayleigh2037
What characteristic of long period comets suggest they come directly from the oort cloud?
Answers: 2

Computers and Technology, 23.06.2019 04:31, hargunk329
Q13 what function does a security certificate perform? a. creates user accounts b. scrambles data c. identifies users d. creates password policies e. provides file access
Answers: 1

Computers and Technology, 23.06.2019 10:00, uwunuzzles
How do i delete my account on this because i didn't read this agreements and also i put age at xd
Answers: 1

Computers and Technology, 25.06.2019 06:10, gabbypittman20
In your pest busters game, how does player 2 move the ship_2 object? a pressing the w and s keys b. pressing the up arrow and down arrow keys c. moving the mouse from side to side d. moving the mouse up and down select the best answer from the choices provided
Answers: 3
You know the right answer?
Given the Solid class, extend it with:
Pyramid
Cylinder
RectangularPrism
Spher...
Cylinder
RectangularPrism
Spher...
Questions in other subjects:


Mathematics, 22.06.2019 19:00


History, 22.06.2019 19:00




History, 22.06.2019 19:00
