
Computers and Technology, 19.08.2020 20:01 ThetPerson
Need help to complete C++ assignment to write simple hash function :
Instructions : This insert function should compute a good hash function of value. This hash function should return the least-significant three decimal digits (a number from 0 to 999) of the variable value. This hash should be used as an index into the thousand-element vector table that has been initialized with -1 in each element. If the element at this location of table is available (currently set to -1), you can replace the element with value. If this location is not available (currently set to some other value than -1) then you should check the next element, repeatedly, until you find an available element and can store value there. The insert() function should then return the number of times a location in the hash table was identified to store value but was not available.
Assignment :
#include
#include
#include
#include
#include
int insert(int value, std::vector &table) {
// Code to insert value into a hashed location in table
// where table is a vector of length 1000.
// Returns the number of collisions encountered when
// trying to insert value into table.
}
int main() {
int i, j, hit, max_hit = 0, max_value = -1;
std::vector value(500);
int old_value = 0;
for (i = 0; i < 500; i++) {
old_value += rand()%100;
value[i] = old_value;
}
// create hash table of size 1000 initialized with -1
std::vector table(1000,-1);
for (i = 0; i < 500; i++) {
hit = insert(value[i],table);
if (hit > max_hit) {
max_hit = hit;
max_value = value[i];
}
}
std::cout << "Hashing value " << max_value << " experienced " << max_hit << " collisions." << std::endl <
for (j = 0; j < 1000; j += 10) {
std::cout << std::setw(3) << j << ":";
for (i = 0; i < 10; i++) {
if (table[j+i] == -1)
std::cout << " ";
else
std::cout << std::setw(6) << table[j+i];
}
std::cout << std::endl;
}
return 0;
}

Answers: 1

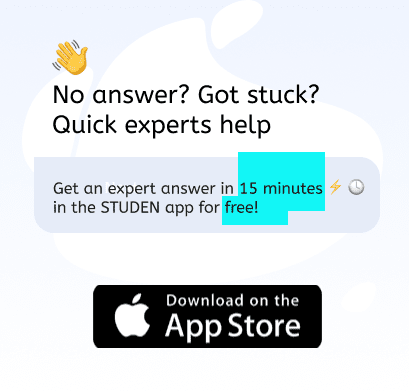
Other questions on the subject: Computers and Technology

Computers and Technology, 22.06.2019 01:30, yudayang2012pa9u8p
Consider the following statements: #include #include class temporary { private: string description; double first; double second; public: temporary(string = "", double = 0.0, double = 0.0); void set(string, double, double); double manipulate(); void get(string& , double& , double& ); void setdescription(string); void setfirst(double); void setsecond(double); }; write the definition of the member function set() so that the instance variables are set according to the parameters. write the definition of the constructor so that it initializes the instance variables using the function set() write the definition of the member function manipulate() that returns a decimal number (double) as follows: if the value of description is "rectangle", it returns first * second if the value of description is "circle" it returns the area of a circle with radius first if the value of description is "cylinder" it returns the volume of a cylinder with radius first and height second. hint: the volume of a cylinder is simply the area of the circle at the base times the height. if the value of description is "sphere" it returns the volume of the sphere with radius first. otherwise it returns -1.0;
Answers: 1

Computers and Technology, 22.06.2019 05:30, donmak3833
Agood flowchart alludes to both the inputs and outputs you will need to receive and give to the user. true or false?
Answers: 3

Computers and Technology, 23.06.2019 00:30, lilobekker5219
Knowing that the central portion of link bd has a uniform cross sectional area of 800 mm2 , determine the magnitude of the load p for which the normal stress in link bd is 50 mpa. (hint: link bd is a two-force member.) ans: p = 62.7 kn
Answers: 2

Computers and Technology, 23.06.2019 20:30, cristalcastro901
If an appliance consumes 500 w of power and is left on for 5 hours, how much energy is used over this time period? a. 2.5 kwh b. 25 kwh c. 250 kwh d. 2500 kwh
Answers: 1
You know the right answer?
Need help to complete C++ assignment to write simple hash function :
Instructions : This insert fun...
Questions in other subjects:


Arts, 15.04.2020 23:05


Mathematics, 15.04.2020 23:05



Mathematics, 15.04.2020 23:05


Mathematics, 15.04.2020 23:05

Mathematics, 15.04.2020 23:05