
Computers and Technology, 18.06.2020 02:57 kathyc53
Write a class named GessGame for playing an abstract board game called Gess. Rules below. Note that when a piece's move causes it to overlap stones, any stones covered by the footprint get removed, not just those covered by one of the piece's stones. It is not legal to make a move that leaves you without a ring. It's possible for a player to have more than one ring. A player doesn't lose until they have no remaining rings. Locations on the board will be specified using columns labeled a-t and rows labeled 1-20, with row 1 being the Black side and row 20 the White side. The actual board is only columns b-s and rows 2-19. The center of the piece being moved must stay within those boundaries. An edge of the piece may go into columns a or t, or rows 1 or 20, but any pieces there are removed at the end of the move. Black goes first. Probably the easiest way of representing the board is to use a list of lists. You're not required to print the board, but you will probably find it very useful for testing purposes. Your GessGame class must include the following:An init method that initializes any data members. A method called get_game_state that takes no parameters and returns 'UNFINISHED', 'BLACK_WON' or 'WHITE_WON'.A method called resign_game that lets the current player concede the game, giving the other player the win. A method called make_move that takes two parameters - strings that represent the center square of the piece being moved and the desired new location of the center square. For example, make_move('b6', 'e9'). If the indicated move is not legal for the current player, or if the game has already been won, then it should just return False. Otherwise it should make the indicated move, remove any captured stones, update the game state if necessary, update whose turn it is, and return True. Feel free to add whatever other classes, methods, or data members you want. All data members must be private. Whether you think of the array indices as being [row][column] or [column][row] doesn't matter as long as you're consistent. Here's a very simple example of how the class could be used:game = GessGame()move_result = game. make_move('e3', 'e6')game. make_move('e14', 'g14')state = game. get_game_state()game. resign_game()The file must be named: GessGame. py

Answers: 2

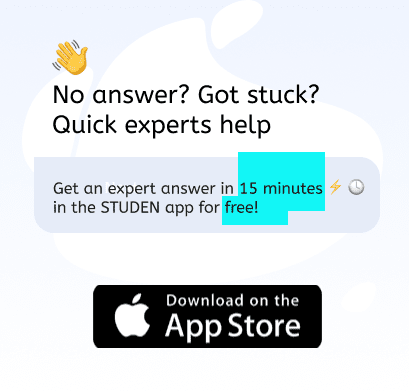
Other questions on the subject: Computers and Technology

Computers and Technology, 23.06.2019 03:30, patience233
Many everyday occurrences can be represented as a binary bit. for example, a door is open or closed, the stove is on or off, and the fog is asleep or awake. could relationships be represented as a binary value? give example.
Answers: 1



Computers and Technology, 25.06.2019 08:50, mountassarhajar2008
The purpose of this problem is to write some small functions and practice passing things around amoung functions.1) the main function shall ask the user to enter three numbers and read the three numbers.2) write a function named findsum that takes three numbers as arguments and returns their sum. the main function shall call this function.3) write a function named findaverage that takes the sum and the number of numbers and returns the average. the main function shall call this function.4) write a function named findsmallest that takes the three numbers and returns the smallest value. the main function shall call this function.5) the main function shall print the results in the following format, with two decimal positions and the decimal points aligned:
Answers: 3
You know the right answer?
Write a class named GessGame for playing an abstract board game called Gess. Rules below. Note that...
Questions in other subjects:




Computers and Technology, 18.09.2019 02:50


Biology, 18.09.2019 02:50

Mathematics, 18.09.2019 02:50



English, 18.09.2019 02:50