
Computers and Technology, 05.06.2020 16:00 alsk101
In this warm up project, you are asked to write a C++ program proj1.cpp that lets the user or the computer play a guessing game. The computer randomly picks a number in between 1 and 100, and then for each guess the user or computer makes, the computer informs the user whether the guess was too high or too low. The program should start a new guessing game when the correct number is selected to end this run (see the sample run below).
Check this example and see how to use functions srand(), time() and rand() to generate the random number by computer.
Example of generating and using random numbers:
#include
#include // srand and rand functions
#include // time function
using namespace std;
int main()
{
const int C = 10;
int x;
srand(unsigned(time(NULL))); // set different seeds to make sure each run of this program will generate different random numbers
x = ( rand() % C);
cout << x << endl;
x = ( rand() % C ); // generate a random integer between 0 and C-1
cout << x << endl;
return 0;
}
Here is a sample run of the program:
Would you like to (p)lay or watch the (c)omputer play?
p
Enter your guess in between 1 and 100.
45
Sorry, your guess is too low, try again.
Enter your guess in between 1 and 100.
58
Sorry, your guess is too low, try again.
Enter your guess in between 1 and 100.
78
Sorry, your guess is too high, try again.
Enter your guess in between 1 and 100.
67
Sorry, your guess is too low, try again.
Enter your guess in between 1 and 100.
70
Sorry, your guess is too high, try again.
Enter your guess in between 1 and 100.
68
Sorry, your guess is too low, try again.
Enter your guess in between 1 and 100.
69
Congrats, you guessed the correct number, 69.
Would you like to (p)lay or watch the (c)omputer play or (q)uit?
c
The computer's guess is 50.
Sorry, your guess is too high, try again.
The computer's guess is 25.
Sorry, your guess is too high, try again.
The computer's guess is 13.
Sorry, your guess is too low, try again.
The computer's guess is 19.
Sorry, your guess is too high, try again.
The computer's guess is 16.
Sorry, your guess is too low, try again.
The computer's guess is 17.
Congrats, you guessed the correct number, 17.
Would you like to (p)lay or watch the (c)omputer play or (q)uit?
q
Press any key to continue
So far I was able to create the user input ortion of the program, I need help coding the computer generated guessing game,
if the users input choice of computer at the end or choice of play.. if you could help me build on what I have below greatly appreciate it!!! thank you
code I have got so far:
#include
#include
#include
int main(void) {
srand(time(NULL)); // To not have the same numbers over and over again.
while(true) { // Main loop.
// Initialize and allocate.
int number = rand() % 99 + 2; // System number is stored in here.
int guess; // User guess is stored in here.
int tries = 0; // Number of tries is stored here.
char answer; // User answer to question is stored here.
//std::cout << number << "\n"; // Was used for debug...
while(true) { // Get user number loop.
// Get number.
std::cout << "Enter a number between 1 and 100 (" << 20 - tries << " tries left): ";
std::cin >> guess;
std::cin. ignore();
// Check is tries are taken up.
if(tries >= 20) {
break;
}
// Check number.
if(guess > number) {
std::cout << "Too high! Try again.\n";
} else if(guess < number) {
std::cout << "Too low! Try again.\n";
} else {
break;
}
// If not number, increment tries.
tries++;
}
// Check for tries.
if(tries >= 20) {
std::cout << "You ran out of tries!\n\n";
} else {
// Or, user won.
std::cout<<"Congratulations!! " << std::endl;
std::cout<<"You got the right number in " << tries << " tries!\n";
}
while(true) { // Loop to ask user is he/she would like to play again.
// Get user response.
std::cout << "Would you like to play again (Y/N)? ";
std::cin >> answer;
std::cin. ignore();
// Check if proper response.
if(answer == 'n' || answer == 'N' || answer == 'y' || answer == 'Y') {
break;
} else {
std::cout << "Please enter \'Y\' or \'N\'...\n";
}
}
// Check user's input and run again or exit;
if(answer == 'n' || answer == 'N') {
std::cout << "Thank you for playing!";
break;
} else {
std::cout << "\n\n\n";
}
}
// Safely exit.
std::cout << "\n\nEnter anything to exit. . . ";
std::cin. ignore();
return 0;
}

Answers: 2

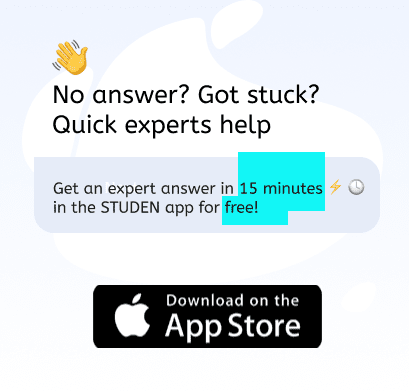
Other questions on the subject: Computers and Technology

Computers and Technology, 23.06.2019 19:30, bbgirl8638
Of the following pieces of information in a document, for which would you most likely insert a mail merge field?
Answers: 3

Computers and Technology, 24.06.2019 12:30, coursonianp8izbc
Do you think media is stereotype ? and why?
Answers: 1

Computers and Technology, 24.06.2019 13:30, lovecats12
To move an excel worksheet tab, simply right-click on it drag and drop it double-click on it delete it
Answers: 1
You know the right answer?
In this warm up project, you are asked to write a C++ program proj1.cpp that lets the user or the co...
Questions in other subjects:








