
Computers and Technology, 23.05.2020 19:59 erikamaldonado661
Task 1 (40 pts). Implement the Quick Sort algorithm as discussed in Lecture 4. (Hint: use the function checked_sorted to check if your output is indeed sorted.) • Task 2 (40 pts). Implement the Counting Sort algorithm as discussed in Lecture 4. (Hint: use the function checked sorted to check if your output is indeed sorted.)package sorting;
import java. util.*;
public class Sort3 {
public static int[] quick_sort (int[] array, int p, int r) {
}
public static int partition (int[] array, int p, int r) {
}
public static int[] counting_sort (int[] array, int k) {
}
public static int[] generate_random_array (int n, int k) {
List list;
int[] array;
Random rnd;
rnd = new Random(System. currentTimeMillis());
list = new ArrayList ();
for (int i = 1; i <= n; i++)
list. add(new Integer(rnd. nextInt(k+1)));
Collections. shuffle(list, rnd);
array = new int[n];
for (int i = 0; i < n; i++)
array[i] = list. get(i).intValue();
return array;
}
public static int[] generate_random_array (int n) {
List list;
int[] array;
list = new ArrayList ();
for (int i = 1; i <= n; i++)
list. add(new Integer(i));
Collections. shuffle(list, new Random(System. currentTimeMillis()));
array = new int[n];
for (int i = 0; i < n; i++)
array[i] = list. get(i).intValue();
return array;
}
/*
* Input: an integer array
* Output: true if the array is acsendingly sorted, otherwise return false
*/
public static boolean check_sorted (int[] array) {
for (int i = 1; i < array. length; i++) {
if (array[i-1] > array[i])
return false;
}
return true;
}
public static void print_array (int[] array) {
for (int i = 0; i < array. length; i++)
System. out. print(array[i] + ", ");
System. out. println();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
int k = 10000;
System. out. println("Quick sort starts ");
for (int n = 100000; n <= 1000000; n=n+100000) {
int[] array = Sort3.generate_random_array(n);
long t1 = System. currentTimeMillis();
array = Sort3.quick_sort(array, 0, n-1);
long t2 = System. currentTimeMillis();
long t = t2 - t1;
boolean flag = Sort3.check_sorted(array);
System. out. println(n + "," + t + "," + flag);
}
System. out. println("Quick sort ends ");
System. out. println("Counting sort starts ");
for (int n = 100000; n <= 1000000; n=n+100000) {
int[] array = Sort3.generate_random_array(n, k);
long t1 = System. currentTimeMillis();
array = Sort3.counting_sort(array, k);
long t2 = System. currentTimeMillis();
long t = t2 - t1;
boolean flag = Sort3.check_sorted(array);
System. out. println(n + "," + t + "," + flag);
}
System. out. println("Counting sort ends ");
}
}

Answers: 3

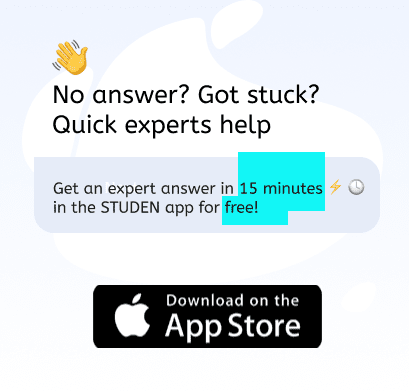
Other questions on the subject: Computers and Technology


Computers and Technology, 23.06.2019 16:30, isaiahhuettnerowgg8d
What is one reason why indoor air pollution has become an increasing problem.
Answers: 1

Computers and Technology, 23.06.2019 19:00, jacobbecker99
Choose the correct citation for the case which established the "minimum contacts" test for a court's jurisdiction in a case. select one: a. brown v. board of education of topeka, 347 u. s. 483 (1954). b. international shoe co. v. washington, 326 u. s. 310 (1945) c. haynes v. gore, 531 u. s. 98 (2000). d. international shoe co. v. washington, 14 u. s. code 336.
Answers: 1

Computers and Technology, 23.06.2019 20:50, terryhgivens5349
3.11.3 quiz: comparing and analyzing function typesquestion 4 of 102 pointswhat can you say about the y-values of the two functions f(x) = 3x2-3 andg(x)=2* - 3?
Answers: 2
You know the right answer?
Task 1 (40 pts). Implement the Quick Sort algorithm as discussed in Lecture 4. (Hint: use the functi...
Questions in other subjects:



Mathematics, 13.11.2020 17:30

Social Studies, 13.11.2020 17:30


English, 13.11.2020 17:30



Mathematics, 13.11.2020 17:30