Push Counter Example (pages 9-12):
//PushCounter. java
import javax. swing....

Computers and Technology, 10.04.2020 04:59 weeblordd
Push Counter Example (pages 9-12):
//PushCounter. java
import javax. swing. JFrame;
public class PushCounter {
public static void main(String[] args) {
JFrameframe = new JFrame("PushCounter");
frame. setDefaultCloseOperation(JFrame. EXIT_ON_CLOSE);
PushCounterPanelpanel = new PushCounterPanel();
frame. getContentPane().add(panel);
frame. pack();
frame. setVisible(true);
}
}
//PushCounterPanel. java
import java. awt.*;
import java. awt. event.*;
import javax. swing.*;
public class PushCounterPanelextends JPanel {
private intcount;
private JButtonpush;
private JLabellabel;
public PushCounterPanel() {
count = 0;
push = new JButton("Push Me!");
label = new JLabel();
push. addActionListener(newButtonListener ());
add(push);
add(label);
setBackground(Color. cyan);
setPreferredSize(newDimension(300, 40)); }
private class ButtonListenerimplements ActionListener {
public void actionPerformed(ActionEventevent){< br />
count++;
label. setText("Pushes: " + count);
}
}
}
Consider "Push Counter" example from GUI lecture slides (pages 9 – 12). Copy the code to your project and run it (note: you’ll only need 2 source files – one for main, and one for panel class; nested listener class DOES NOT require an additional source file).
Update the following example, so that it has an additional button called "Reset counter". When pressed, this button should reset the counter. Note: you may want to be able to determine the event source in your listener. To implement this, use "Determining event sources" example from the slides (pages 20 – 24), so that your listener looks similar to this:
public void actionPerformed(ActionEvent event)
{
if (event. getSource() == left)
label. setText("Left");
else
label. setText("Right");
}
Determining Event Sources Example(pages 20-24):
- The LeftRightPanel class creates one listener and applies it to both buttons.
- When either button is pressed, the actionPerformed method of the listener is invoked.
- The getSource method returns a referenced to the component that generated the event.
-We could have created two listener classes. Then the actionPerformed method would not have to determine where the event is originating.
//LeftRight. java
import javax. swing. JFrame;
public class LeftRight {
public static void main (String[] args) {
JFrame frame = new JFrame("Left Right");
frame. setDefaultCloseOperation(JFrame. EXIT_ON_CLOSE);
frame. getContentPane().add(new LeftRightPanel());
frame. pack();
frame. setVisible(true);
}
}
//LeftRightPanel. java
import java. awt.*;
import java. awt. event.*;
import javax. swing.*;
public class LeftRightPanel extends JPanel {
private Button left, right;
private JLabel label;
private JPanel buttonPanel;
public LeftRightPanel() {
left = new JButton("Left");
right = new JButton("Right");
ButtonListener listener = new ButtonListener();
left. addActionListener(listener);
right. addActionListener(listener);
label = new JLabel("Push a button");
buttonPanel = new JPanel();
buttonPanel. setPreferredSize(new Dimension(200, 40));
buttonPanel. setBackground(Color. blue);
buttonPanel. add(left);
buttonPanel. add(right);
setPreferredSize(new Dimension(200, 80));
setBackground(Color. cyan);
add(label);
add(buttonPanel);
}
private class ButtonListener implements ActionListener {
public void actionPerformed(ActionEvent event) {
if (event. getSource() == left)
label. setText("Left");
else
label. setText("Right");
}
}
}

Answers: 3

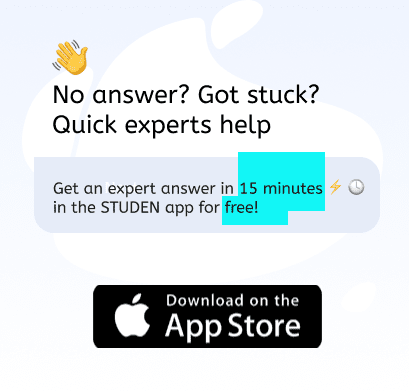
Other questions on the subject: Computers and Technology


Computers and Technology, 24.06.2019 05:30, kaylaamberd
Why is hard disk space important to an audio engineer? why are usb ports and firewire ports useful for an audio engineer? explain in 2-3 sentences. (3.0 points) here's a list of different audio software: ableton live apple inc.'s garageband apple inc.'s logic studio digidesign's pro tools propellerhead sofware's reason sony creative software's acid pro steinberg cubase steinberg nuendo choose one of the software programs listed above, and then go to that software program's web site. read about what the software program is used for, and then write 4-5 sentences about what you learned. (10.0 points) which type of software license is the most limiting? why? explain in 2-3 sentences. (3.0 points) when sending a midi channel voice message, how can you control the volume of the sound? explain in 2-3 sentences. (4.0 points)
Answers: 1

Computers and Technology, 24.06.2019 08:20, bob4059
Evaluate the scenario below and indicate how to handle the matter appropriately. situation: michael received an e-mail from what he thought was his doctor’s office, requesting his social security number. since he had just been in to see his doctor last week, he replied to the e-mail with his social security number.
Answers: 2
You know the right answer?
Questions in other subjects:



Mathematics, 03.11.2020 03:40

Social Studies, 03.11.2020 03:40

History, 03.11.2020 03:40

Mathematics, 03.11.2020 03:40

Mathematics, 03.11.2020 03:40

Mathematics, 03.11.2020 03:40


English, 03.11.2020 03:40