Objective:
To write a C program that calculates the average CPI, total processing time,...

Computers and Technology, 03.03.2020 00:52 sarahsompayrac
Objective:
To write a C program that calculates the average CPI, total processing time, and MIPS of a sequence of instructions, given the number of instruction classes, the CPI and total count of each instruction type, and the clock rate (frequency) of a particular machine.
Inputs:
Number of instruction classes (types)
CPI of each type of instruction
Total instruction count of each type of instruction
Clock rate of machine (MHz)
Output:
Table of parameters
Table of performance (Average CPI, CPU time (ms), MIPS)
Specification:
The program calculates the output based on choosing from a menu of choices, where each choice calls the appropriate procedure, where the choices are:
1) Enter parameters
2) Print table of parameters
3) Print table of performance
4) Quit
Notes:
Make sure all calculations are displayed truncated to 2 decimal fractional places, using the format "%.2f" in the printf statements.
Be sure that execution time is measured in milliseconds (msec).
To typecast an int x to a float y, use y = (float)x orsimply y = 1.0*x
To create proper spacing, use "\t" to tab.
Feel free to use the template "skeleton" code provided {beware: it might not compile correctly until modified appropriately}
#include
#include
/* NOTE: throughout the code, change the name of each procedure or function to something else in order to compile */
/* declare global var's */
//
/* define functions to calculate average cpi, cpu time, and mips */
float "FUNCTION TO CALCULATE AVERAGE CPI"()
{
/* declare local var's, calculate and return average cpi */
}
float "FUNCTION TO CALCULATE CPU TIME"()
{
/* declare local var's, calculate and return cpu time */
}
float "FUNCTION TO CALCULATE MIPS"()
{
/* declare local var's, calculate and return mips */
}
//
void "OPTION #1"()
{
/* declare local var's */
/* Prompt for number of instruction classes and frequency of machine */
/* allocate memory for dynamic arrays for cpi and instruction count */
/* for each instruction class, prompt for CPI and instruction count */
/* update total number of instructions and cycles */
}
//
void "OPTION #2"()
{
/* declare local var's */
/* print table of parameters inputed from OPTION #1, with each row containing the class number, the CPI of the class, and the instruction count of the class, following the sample output on the handout */
}
//
void "OPTION #3"()
{
/* declare local var's */
/* print table of calculations, including the average CPI, the CPU time, and the MIPS */
}
//
int main()
{
/* declare local var's */
/* until user chooses "4", loop */
/* print out menu list */
/* prompt for selection & choose appropriate procedure using either a case statement of if-else if-else statements */
}
Sample Input/Output
Performance assessment:
1) Enter parameters
2) Print table of parameters
3) Print table of performance
4) Quit
Enter selection: 1
Enter the number of instruction classes: 3
Enter the frequency of the machine (MHz): 200
Enter CPI of class 1: 2
Enter instruction count of class 1 (millions): 3
Enter CPI of class 2: 4
Enter instruction count of class 2 (millions): 5
Enter CPI of class 3: 6
Enter instruction count of class 3 (millions): 7
Performance assessment:
1) Enter parameters
2) Print table of parameters
3) Print table of performance
4) Quit
Enter selection: 2
|Class |CPI |Count |
|1 |2 |3 |
|2 |4 |5 |
|3 |6 |7 |
Performance assessment:
1) Enter parameters
2) Print table of parameters
3) Print table of performance
4) Quit
Enter selection: 3
|Performance |Value |
|Average CPI |4.53 |
|CPU Time (ms) |340.00 |
|MIPS |44.12 |
Performance assessment:
1) Enter parameters
2) Print table of parameters
3) Print table of performance
4) Quit
Enter selection: 4

Answers: 2

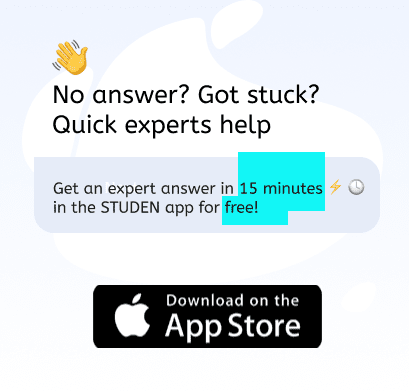
Other questions on the subject: Computers and Technology


Computers and Technology, 22.06.2019 08:30, ruddymorales1123
Linda subscribes to a cloud service. the service provider hosts the cloud infrastructure and delivers computing resources over the internet. what cloud model is linda using
Answers: 1

Computers and Technology, 24.06.2019 07:00, jordaaan101
Guys do you know sh27 cause he hacked me : ( pidgegunderson my old user
Answers: 2

Computers and Technology, 24.06.2019 08:30, ladybuggirl400
@josethesolis i need can anyone text me and follow me
Answers: 1
You know the right answer?
Questions in other subjects:

Mathematics, 28.12.2020 22:50

Health, 28.12.2020 22:50








Mathematics, 28.12.2020 22:50