Hlp
'''
math_funcs
complete the following functions. you must, must,...

Computers and Technology, 11.10.2019 20:20 genyjoannerubiera
Hlp
'''
math_funcs
complete the following functions. you must, must, must add:
1) a doc comment in the proper location for each function that describes
the basic purpose of the function.
2) at least 5 doc test comments in each function that:
* test that the function does what it is supposed to do
* tests that it does what it's supposed to do with odd inputs
* tests "edge" cases (numbers at, just above, just below min/max, empty strings, etc.)
you must, must, must then test each of your methods by both:
1) running the "main. py" script
2) running this module directly to run the doc tests
except as noted, you can implement the functions however you like. and if your grade-school
math's out of date, google's your friend for formulas (but not for code).
challenge: use try/except blocks to avoid crashes when passing in unexpected parameters.
circle_area
return the area of a circle with a radius supplied by the parameter.
note that you must use the "pi" constant from the math module, so
use an import statement. if the radius passed is less than 1 or
greater than 1000, print "error" and return 0.
sphere_surface_area
return the surface area of a sphere with the supplied radius. slightly
different error check here: if the radius passed is less than 1 or
greater than 250, print "error" and return 0.
sphere_volume
return the volume of a sphere with the supplied radius. again, slightly
different error check here: if the radius passed is less than 1 or
greater than 100, print "error" and return 0.
'''
# import that pi constant here
from math import pi
def circle_area(radius):
area = math. pi * radius**2
if radius > 1 or > 1000:
print(error)
return 0
from math import pi
def sphere_surface_area(radius):
sa = 4 * math. pi * radius * 2
if radius < 1 or radius > 250:
print(error)
return 0
from math import pi
def sphere_volume(radius):
sv = 4 * math. pi * radius * 3
if radius < 1 or radius > 100
print(error)
return 0
if __name__ == "__main__":
import doctest
doctest. testmod()

Answers: 3

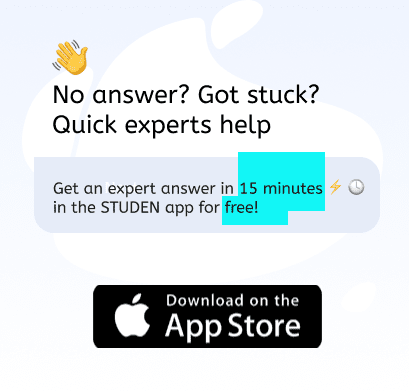
Other questions on the subject: Computers and Technology


Computers and Technology, 22.06.2019 04:30, zetrenne73
How can you know if the person or organization providing the information has the credentials and knowledge to speak on this topic? one clue is the type of web site it is--the domain name ".org" tells you that this site is run by a nonprofit organization.
Answers: 2

Computers and Technology, 22.06.2019 09:00, jgrable5175
Designing a mobile web page is a little different from designing a regular web page. name at least three features that should be considered when designing a website that is mobile phone-friendly, and briefly explain why they are important.
Answers: 1

Computers and Technology, 22.06.2019 14:30, chaparro0512
Create a pseudocode design to prompt a student for their student id and the titles of the three classes they want to add. the solution should display the student’s id and a total bill. • bill a student using the following rules: o students can only add up to 3 classes at a time.
Answers: 3
You know the right answer?
Questions in other subjects:





History, 19.09.2019 13:00

Mathematics, 19.09.2019 13:00

Mathematics, 19.09.2019 13:00


Social Studies, 19.09.2019 13:00