
Advanced Placement (AP), 13.03.2021 05:00 Dweath50
Complete the class DiscountedItem below that inherits from Item and adds an discount instance variable with a constructor, get/set, and a toString method. Uncomment the testing code in main to add discounted items to the cart.
import java. util.*;
/**
The ShoppingCart class has an ArrayList of Items.
You will write a new class DiscountedItem that extends Item.
This code is adapted from https://practiceit. cs. washington. edu/problem/view/bjp4/chapter9/e10- DiscountBill
*/
public class Tester
{
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
cart. add(new Item("bread", 3.25));
cart. add(new Item("milk", 2.50));
// Uncomment these to test
//cart. add(new DiscountedItem("ice cream", 4.50, 1.50));
//cart. add(new DiscountedItem("apples", 1.35, 0.25));
cart. printOrder();
}
}
// DiscountedItem inherits from Item
class DiscountedItem extends Item
{
// add an instance variable for the discount
// Add constructors that call the super constructor
// Add get/set methods for discount
public double getDiscount()
{
return 0.0; // return discount here instead of 0
}
// Add a toString() method that returns a call to the super toString
// and then the discount in parentheses using the super. valueToString() method
}
class ShoppingCart
{
private ArrayList order;
private double total;
private double internalDiscount;
public ShoppingCart()
{
order = new ArrayList ();
total = 0.0;
internalDiscount = 0.0;
}
public void add(Item i) {
order. add(i);
total += i. getPrice();
if (i instanceof DiscountedItem)
internalDiscount += ((DiscountedItem) i).getDiscount();
}
/** printOrder() will call toString() to print */
public void printOrder() {
System. out. println(this);
}
public String toString() {
return discountToString();
}
public String discountToString() {
return orderToString() + "\nSub-total: " + valueToString(total) + "\nDiscount: " + valueToString(internalDiscount) + "\nTotal: " + valueToString(total - internalDiscount);
}
private String valueToString(double value) {
value = Math. rint(value * 100) / 100.0;
String result = "" + Math. abs(value);
if(result. indexOf(".") == result. length() - 2) {
result += "0";
}
result = "$" + result;
return result;
}
public String orderToString() {
String build = "\nOrder Items:\n";
for(int i = 0; i < order. size(); i++) {
build += " " + order. get(i);
if(i != order. size() - 1) {
build += "\n";
}
}
return build;
}
}
class Item {
private String name;
private double price;
public Item()
{
this. name = "";
this. price = 0.0;
}
public Item(String name, double price) {
this. name = name;
this. price = price;
}
public double getPrice() {
return price;
}
public String valueToString(double value) {
String result = "" + Math. abs(value);
if(result. indexOf(".") == result. length() - 2) {
result += "0";
}
result = "$" + result;
return result;
}
public String toString() {
return name + " " + valueToString(price);
}
}

Answers: 1

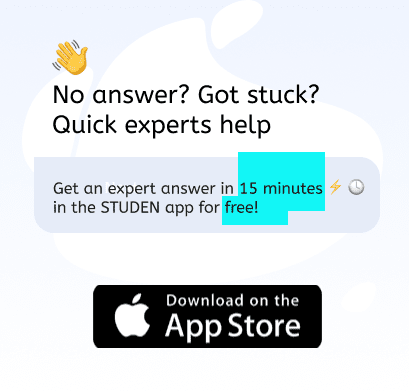
Other questions on the subject: Advanced Placement (AP)

Advanced Placement (AP), 22.06.2019 08:30, joyelewis58
If u were an animal what would it be
Answers: 2


Advanced Placement (AP), 26.06.2019 21:40, tasnimabdallah971
Dr. williams conducted a survey in the mall, measuring the shopping habits of people ages 15, 25, 35, 45, and 55, with 10 people in each group. which of the following best describes her study? an experiment a cross-sectional study a case study a longitudinal study a double-blind study
Answers: 1

Advanced Placement (AP), 28.06.2019 01:30, oct316mb
Amedical coder in a cardiologist's office is preparing a report about clinical services provided to a patient. the report will be sent to the patient's insurance company. the services included an ekg to record the heart beat and an echocardiogram to take pictures of the heart. for every service, the coder will record a(n) procedure code. definition code. who code. icd-o-3 code.
Answers: 1
You know the right answer?
Complete the class DiscountedItem below that inherits from Item and adds an discount instance variab...
Questions in other subjects:



Mathematics, 14.01.2020 20:31




English, 14.01.2020 20:31

